C Programming Language Free Course
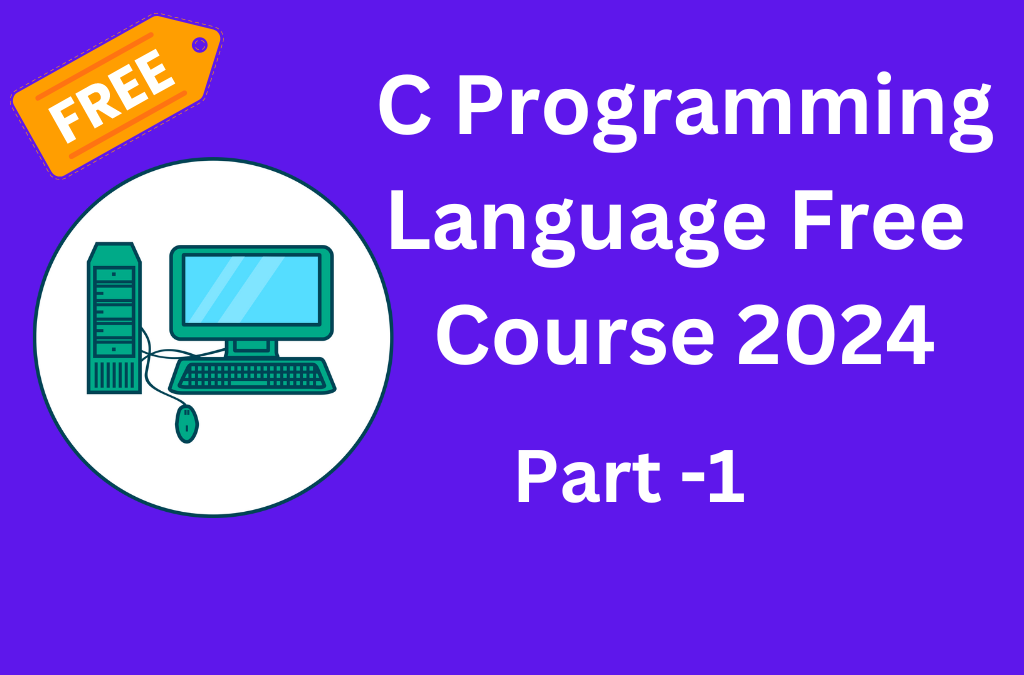
1.History of C Programming
The C programming language is one of the most widely used programming languages and has a rich history rooted in the development of modern computing.
- Origins:
C was developed in the early 1970s by Dennis Ritchie at AT&T Bell Laboratories. It was designed as a successor to the B language, which itself was a simplification of BCPL (Basic Combined Programming Language). - Purpose:
C was initially created to rewrite the UNIX operating system, enabling it to run on different types of hardware with minimal changes. This required a language that was both low-level (close to the hardware) and portable (easy to move from one machine architecture to another). - Features:
C provides control structures, data types, and low-level access to memory. Its ability to manipulate memory addresses directly is one of the reasons it became the language of choice for system-level programming. - Standardization:
The first standardized version of C, known as ANSI C, was developed by the American National Standards Institute (ANSI) in 1989, which led to the term “C89.” It was followed by ISO C in 1990, C99 in 1999, C11 in 2011, and C18 in 2018. These standards added various features to the language, including new data types, library functions, and other improvements.
2. Assembly & Machine Languages
- Machine Language:
Machine language is the lowest level of programming language, consisting of binary code (0s and 1s) that a computer’s central processing unit (CPU) can execute directly. Each type of CPU has its own specific machine language. - Assembly Language:
Assembly language is a step above machine language. It uses mnemonic codes and labels instead of binary, making it easier for humans to understand and write. For example, instead of a binary instruction like10110000
, assembly language might use the mnemonicMOV
to represent moving data between registers. - Assembler:
An assembler is a tool that translates assembly language into machine language. Unlike high-level languages, assembly language is specific to a particular computer architecture.
3. Editors
- Definition:
Editors are software applications used to write and edit code. They are essential tools for programmers and can range from simple text editors to sophisticated integrated development environments (IDEs). -
Examples:
- Simple Editors: Notepad (Windows), TextEdit (Mac)
- Code Editors: Visual Studio Code, Sublime Text, Atom
- IDEs: Eclipse, NetBeans, IntelliJ IDEA
- Features:
Code editors often include syntax highlighting, auto-completion, error detection, and integrated debugging tools, which make coding easier and more efficient.
4. Translators
- Definition:
Translators convert high-level programming code into machine code that can be executed by a computer’s CPU. There are two main types of translators: compilers and interpreters. - Compilers:
A compiler translates the entire program code into machine code before the program is run. This process generates an executable file, which can be run independently of the source code. C is a compiled language. - Interpreters:
An interpreter translates code line-by-line and executes it simultaneously. This means the code needs to be translated every time it runs, which can slow down execution. Languages like Python often use interpreters.
5. Programming Rules
- Consistency:
Writing code in a consistent style, including naming conventions and indentation, improves readability and maintainability. - Clarity:
Code should be clear and understandable, using comments where necessary to explain complex logic. - Modularity:
Code should be organized into functions and modules, allowing for reuse and easier maintenance. - Error Handling:
Programs should anticipate potential errors and handle them gracefully, ensuring the software does not crash unexpectedly.
6. Algorithm
- Definition:
An algorithm is a step-by-step procedure or formula for solving a problem. Algorithms are written in a way that they can be implemented in any programming language. - Characteristics of Algorithms:
- Finiteness: Algorithms must terminate after a finite number of steps.
- Definiteness: Each step must be clear and unambiguous.
- Input and Output: Algorithms may take zero or more inputs and produce one or more outputs.
- Effectiveness: Algorithms should be simple and straightforward.
7. Structure of a C Program
A typical C program consists of the following parts:
- Preprocessor Directives:
Lines beginning with#
, such as#include <stdio.h>
, which include standard libraries or define constants. - Main Function:
Themain()
function is where program execution begins. Every C program must have amain
function.Example:
int main() {
// Code here
return 0;
}
- Variable Declarations:
Before using a variable, it must be declared with a specific data type. - Statements and Expressions:
Instructions that perform operations, assignments, and function calls. - Comments:
Lines of text that are ignored by the compiler and provide explanations to human readers.
8. C Character Set
The character set in C includes the following:
- Letters:
Uppercase (A-Z) and lowercase (a-z) - Digits:
0-9 - Special Characters:
Symbols such as!
,@
,#
,$
,%
,^
,&
,*
,(
,)
,{
,}
,[
,]
,;
,:
,,
,<
,>
,.
,?
,/
,\
,'
,"
,|
,~
,=
,+
,-
- Whitespace Characters:
Space, horizontal tab (\t
), newline (\n
), vertical tab (\v
), form feed (\f
), and carriage return (\r
)
9. Keywords
- Definition:
Keywords are reserved words in C that have special meaning and cannot be used as identifiers. They form the core syntax and structure of the language. -
Examples:
int
,char
,float
,if
,else
,while
,for
,return
,void
,switch
,case
,break
,continue
,typedef
,struct
,union
,enum
,sizeof
,static
,extern
,auto
,register
,const
,volatile
,unsigned
,signed
,long
,short
,goto
10. Identifiers
- Definition:
Identifiers are names given to entities such as variables, functions, arrays, etc. They are created by programmers to give meaningful names to elements in their code. - Rules to Form an Identifier:
- Must begin with a letter (uppercase or lowercase) or an underscore
_
. - Can contain letters, digits, and underscores.
- Cannot be a keyword.
- Case-sensitive (
total
,Total
, andTOTAL
are different identifiers). - No whitespace or special characters allowed.
- Must begin with a letter (uppercase or lowercase) or an underscore
11. Variables, Constants, and Types
- Variables:
Variables are named storage locations in memory, each with a specific data type, where data can be stored and modified.Example:
int age = 25;
- Constants:
Constants are fixed values that do not change during the execution of a program. They are declared using theconst
keyword or#define
.Example:
const float PI = 3.14159;
12. Comments
- Definition:
Comments are non-executable lines in code that help explain what the code does. They are meant for humans to read and understand the code better. - Types:
- Single-line comments: Start with
//
and extend to the end of the line. - Multi-line comments: Start with
/*
and end with*/
. Can span multiple lines.
Example:
// This is a single-line comment
/*
This is a multi-line comment
*/
- Single-line comments: Start with
13. Data Types
- Primary Data Types:
int
: Integer values.char
: Single character.float
: Floating-point numbers (single precision).double
: Double-precision floating-point numbers.
- Derived Data Types:
- Arrays: Collection of elements of the same data type.
- Pointers: Variables that store memory addresses.
- Structures: User-defined data types that group different data types.
- Unions: Similar to structures, but with shared memory for all members.
- Void Type:
Thevoid
type is used to specify that a function does not return a value.
14. Operators
Operators in C are symbols that perform operations on one or more operands.
- Arithmetic Operators:
+
,-
,*
,/
,%
(modulus) - Relational Operators:
==
,!=
,<
,>
,<=
,>=
- Logical Operators:
&&
(AND),||
(OR),!
(NOT) - Bitwise Operators:
&
, `|